Guided Tutorial
The following tutorial will guide you through the process of adding authentication features to a Next.js application that uses a Next.js backend.
#
Architecture OverviewFirst, let's get a high-level overview of how everything is supposed to work.
- Managed service
- Self hosted
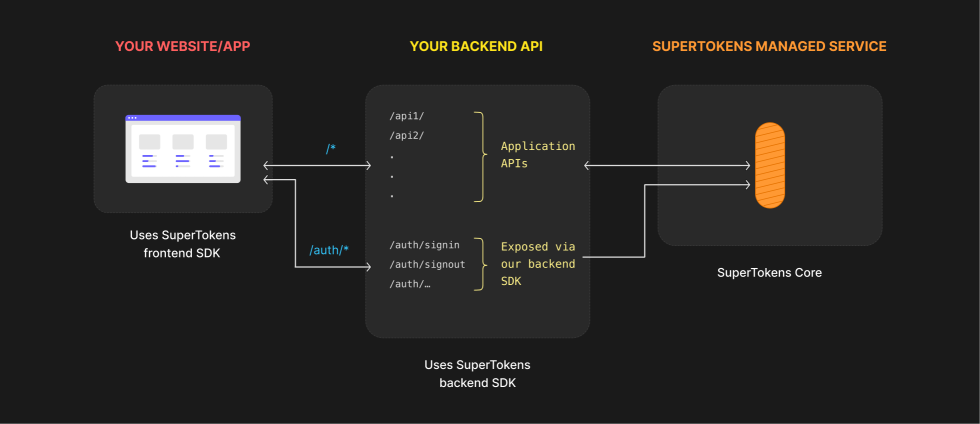
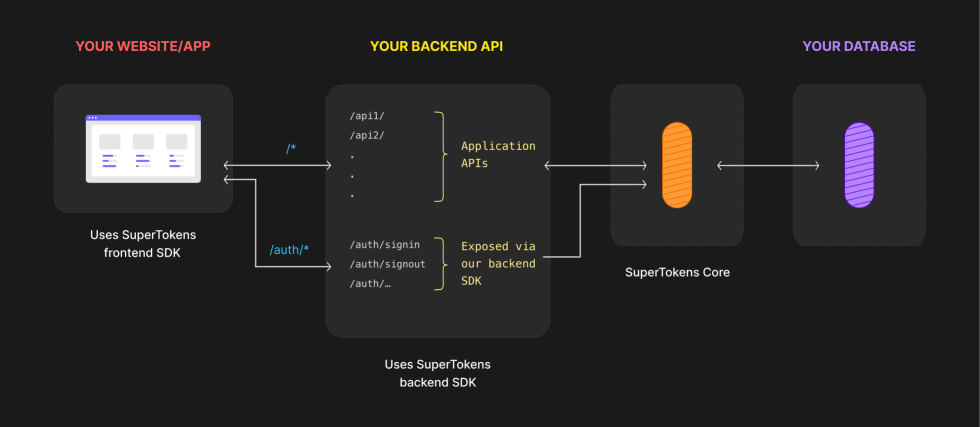
You can directly integrate SuperTokens in your existing services. The authentication routes will be properly exposed based on your configuration.
There are three main components that you need to keep in mind here:
- The Frontend SDK - Provides pre built UI components and functions to manage authentication from your frontend app.
- The Backend SDK - Comunicates with the Core Service and exposes all the auth related APIs for your frontend to call. It also enables session management and access control for your application.
- The SuperTokens Core Service - This is where we persist the state of the authentication functionalities. The service gets called by the backend SDK throughout every exposed feature. You can choose between self hosting the SuperTokens Core Service or using our managed offering.
For more information on how everything works, please check the following page.
#
Project StructureUse our starter project to get up and running quickly. It comes with full config examples based on your selection. Just run the following command to install everything:
npx create-supertokens-app@latest --recipe=emailpassword --frontend=next
The command creates a sample Next.js app with authentication configured to work with SuperTokens. Bellow, we go through the main files that you will need to copy/update based on your setup.
/config
#
appInfo.ts
Includes information about your application. The information configured works for the demo app, but you will likely want to change it based on your setup. You can learn more about this config here.backend.ts
Exports a function that provides configuration instructions for the SuperTokens Backend SDK. Besides theappInfo
that is imported from the previous file, there are a couple of additional things that get configured here:- How the app will connect to the SuperTokens Core Service. The value is set to
https://try.supertokens.com
. This is a demo server we host so you can get started quickly. Later on in the guide, we will spin up a dedicated managed or self hosted core service, and, based on that, we will updated the URL. - What recipes get initialised. Think of recipes as modules or features you enable. The list of recipes that are enabled here will affect which APIs are exposed via the Backend SDK middleware. Based on your use case, you may have to modify the parameters values provided to the recipes. We will go into detail about each recipe in the next sections.
- How the app will connect to the SuperTokens Core Service. The value is set to
frontend.tsx
You can copy and paste this file directly into your existing project without any additional changes. It uses the config values that you defined in theappInfo.ts
file as well as the authentication recipes that you have selected.
/api/auth
#
This folder includes a file that sets up the authentication endpoints. You can copy it into your existing project without any additional changes.
/api/user
#
In here you will find an example of how to expose session related data through an endpoint.
You can notice that each api function makes use of ensureSuperTokensInit
. This way you can make sure that you are initializing the SuperTokens Backend SDK per API call. This is needed since your Next backend may be running in a serverless function, so each API invocation will have to init the Backend SDK.
/auth
#
This folder includes a file that will add the authentication routes for the web client. You can copy and paste this file directly into your existing project without any additional changes.
It will include the prebuilt UI components based on the configuration that was set in the frontend.tsx
file.
layout.tsx
#
Here is where you add the SuperTokensProvider
component over your existing layout. This way we provide the authentication context to all the pages in your app.
The component is defined in the /components/supertokensProvider.tsx
file. It initializes the SuperTokens React SDK and exports the component.
#
RecipesRecipes are the building blocks that you can use to enable different authentication functionalities. In this section we will talk about specific configuration options and guide you through how to manage different login scenarios.
#
Email/Password AuthenticationYou do not have to configure anything in particular for this recipe. The backend and the frontend code already includes the initialization steps in each config files.
Custom UI
If you plan on using your own custom components for the UI you will have to use the supertokens-web-js
library and manually call the authentication routes.
Use this demo app for a guideline on how everything on the backend should work and check our page that shows how authentication methods should look like on the frontend.
#
Session ManagementBesides all the authentication recipes that get initialized in th recipeList
array, you might have noticed another call, Session.init()
.
This enables the session management functionalities that SuperTokens provides for your application.
To protect your api functions, you will have to call the withSession
wrapper function in order to verify the session data. Check the user.ts
file for a full example.
On the frontend the SessionAuth
component can be used as a wrapper over routes that require authentication. If a user has not logged in, they will automatically
get redirected to the public sign in page.
#
SuperTokens Core SetupIn our example app we used try.supertokens.com to host the example app. You can replace the endpoint with your the correct one based on your configuration.
If you want to use our managed service, check this guide on how to sign up and create your environment. Otherwise, for self-hosted instances, you can follow either the Docker guide or the other instructions, for non-docker scenarios.